In this tutorial, you will learn how to make API calls in javascript in 4 different ways.
Contents
- XMLHttpRequest
- JQuery
- Fetch API
- Axios
XML HTTP Request
XMLHttpRequest is one of the javascript global window objects. Before ES6, it is the only way of making API calls. It works in both old and new browsers and it is deprecated in ES6 but still widely used.
var request = new XMLHttpRequest();
request.open('GET', 'https://jsonplaceholder.typicode.com/todos')
request.send();
request.onload = ()=>{
console.log(JSON.parse(request.response));
}
jQuery Ajax
ajax method in jQuery is used to perform asyncronous API calls. To use jQuery, we need to include the jQuery script file to access its methods.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
$(document).ready(function(){
$.ajax({
url: "https://jsonplaceholder.typicode.com/todos",
type: "GET",
success: function(result){
console.log(result);
}
})
})
Fetch
fetch is similar to XMLHttpRequest but here we can make async calls in a simpler way. It returns the promise and .then() method is used to perform the action on the response. Fetch is only supported in modern browsers and doesn’t work in old browsers.
fetch('https://jsonplaceholder.typicode.com/todos').then(response =>{
return response.json();
}).then(data =>{
console.log(data);
})
Axios
Axios is one of the popular open-source libraries to make API calls. We can also include it as a script in an HTML file to make API calls. It also returns a promise as fetch API.
It is one of the modern and preferred by most developers to make HTTP requests.
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
axios.get("https://jsonplaceholder.typicode.com/todos")
.then(response =>{
console.log(response.data)
})
JavaScript API Calls Cheatsheet
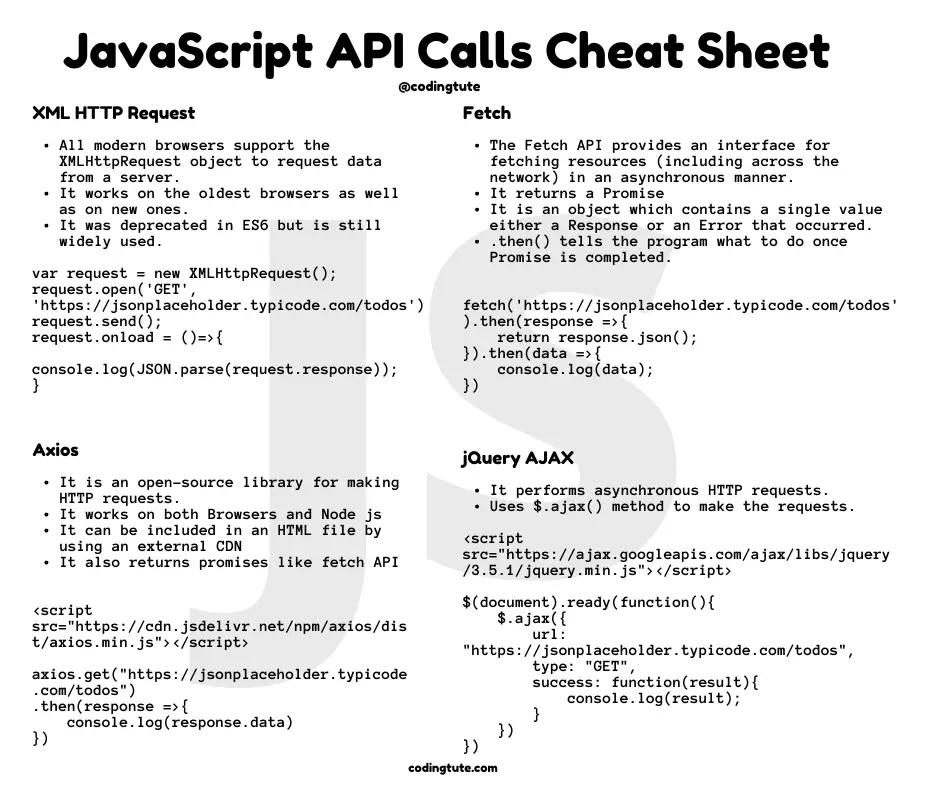
Follow us on Facebook, YouTube, Instagram, and Twitter for more exciting content and the latest updates.