In this example, you will learn to find the roots of a quadratic equation.
A quadratic equation will have two roots and a standard quadratic equation is defined as ax2 + bx + c = 0 where a,b and c are real numbers and a!=0.
The nature of the quadratic equation is determined by the discriminant value (b2 – 4ac).
- If discriminant value is greater than 0, then the roots are real and different.
- If discriminant value is equal to 0, then the roots are real and equal.
- If discriminant value is less than 0, then the roots are complex and different.
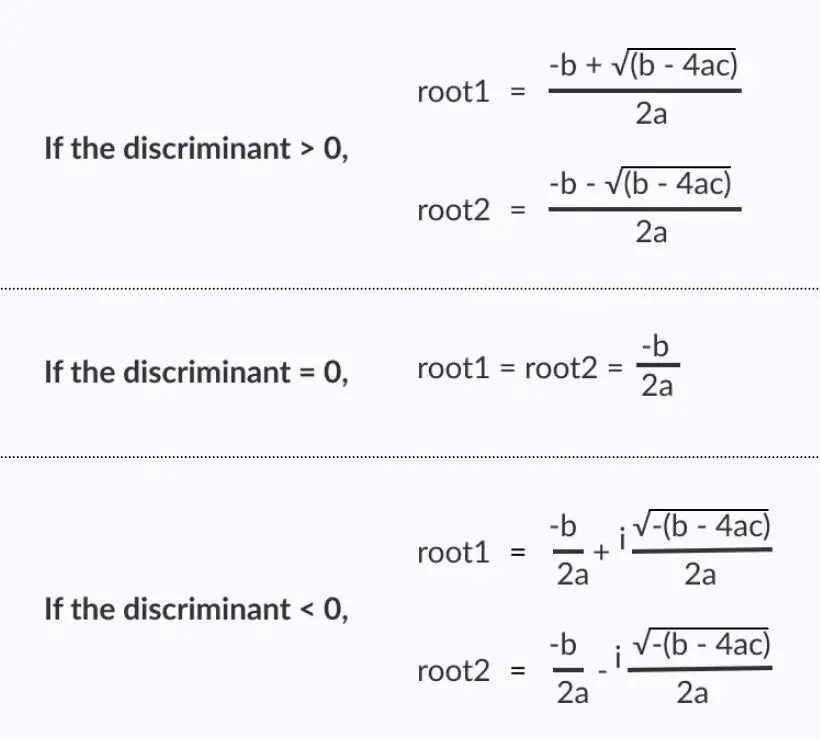
C Program to Find the Roots of a Quadratic Equation
#include <stdio.h>
#include <math.h>
int main() {
float a, b, c, root1, root2, discriminant, realPart, imagPart;
printf("Enter coefficients a, b and c: ");
scanf("%f %f %f", &a, &b, &c);
// calculate discriminant
discriminant = b*b - 4*a*c;
// condition for roots are real and different
if (discriminant > 0) {
root1 = (-b + sqrt(discriminant)) / (2 * a);
root2 = (-b - sqrt(discriminant)) / (2 * a);
printf("Root1 = %.3f and Root2 = %.3f", root1, root2);
}
// condition for roots are real and equal
else if (discriminant == 0) {
root1 = root2 = -b / (2 * a);
printf("Root1 = Root2 = %.3f;", root1);
}
// if roots are complex
else {
realPart = -b / (2 * a);
imagPart = sqrt(-discriminant) / (2 * a);
printf("Root1 = %.3f+%.3fi and Root2 = %.3f-%.3fi", realPart, imagPart, realPart, imagPart);
}
return 0;
}
Output
Enter coefficients a, b and c: 1 1 4
Root1 = -0.500+1.936i and Root2 = -0.500-1.936i
Follow us on Facebook, YouTube, Instagram, and Twitter for more exciting content and the latest updates.